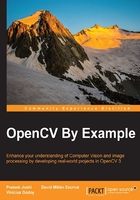
Managing dependencies
CMake has the ability to search our dependencies and external libraries, giving us the facility to build complex projects depending on external components in our projects and by adding some requirements.
In this book, the most important dependency is, of course, OpenCV, and we will add it to all our projects:
cmake_minimum_required (VERSION 2.6) cmake_policy(SET CMP0012 NEW) PROJECT(Chapter2) # Requires OpenCV FIND_PACKAGE( OpenCV 3.0.0 REQUIRED ) # Show a message with the opencv version detected MESSAGE("OpenCV version : ${OpenCV_VERSION}") include_directories(${OpenCV_INCLUDE_DIRS}) link_directories(${OpenCV_LIB_DIR}) # Create a variable called SRC SET(SRC main.cpp ) # Create our executable ADD_EXECUTABLE( ${PROJECT_NAME} ${SRC} ) # Link our library TARGET_LINK_LIBRARIES( ${PROJECT_NAME} ${OpenCV_LIBS} )
Now, let's understand the working of the script:
cmake_minimum_required (VERSION 2.6) cmake_policy(SET CMP0012 NEW) PROJECT(Chapter2)
The first line defines the minimum CMake version; the second line tells CMake to use the new behavior of CMake so that it can correctly recognize numbers and Booleans constants without dereferencing variables with such names. This policy was introduced in CMake 2.8.0, and CMake warns when the policy is not set to version 3.0.2. Finally, the last line defines the project title:
# Requires OpenCV FIND_PACKAGE( OpenCV 3.0.0 REQUIRED ) # Show a message with the opencv version detected MESSAGE("OpenCV version : ${OpenCV_VERSION}") include_directories(${OpenCV_INCLUDE_DIRS}) link_directories(${OpenCV_LIB_DIR})
This is where we search for our OpenCV dependency. FIND_PACKAGE
is the function that allows us to find our dependencies and the minimum version required if this dependency is required or optional. In this sample script, we look for OpenCV in version 3.0.0 or greater and it is a required package.
Note
The FIND_PACKAGE
command includes all OpenCV submodules, but you can specify the submodules that you want to include in the project by making your application smaller and faster. For example, if we are going to work only with the basic OpenCV types and core functionalities, we can use the following command:
FIND_PACKAGE(OpenCV 3.0.0 REQUIRED core)
If CMake does not find it, it returns an error and does not prevent us from compiling our application.
The MESSAGE function shows a message on the terminal or CMake GUI. In our case, we will show the OpenCV version, as follows:
OpenCV version : 3.0.0
${OpenCV_VERSION}
is a variable where CMake stores the OpenCV package version.
The include_directories()
and link_directories()
add the header and the directory of the specified library to our environment. OpenCV's CMake module saves this data in the ${OpenCV_INCLUDE_DIRS}
and ${OpenCV_LIB_DIR}
variables. These lines are not required in all platforms, such as Linux, because these paths are normally in the environment, but it's recommended that you have more than one OpenCV version to choose from the correct link and include directories:
# Create a variable called SRC SET(SRC main.cpp ) # Create our executable ADD_EXECUTABLE( ${PROJECT_NAME} ${SRC} ) # Link our library TARGET_LINK_LIBRARIES( ${PROJECT_NAME} ${OpenCV_LIBS} )
This last line creates the executable and links it to the OpenCV library, as we saw in the previous section, Creating a library.
There is a new function in this piece of code called SET
. This function creates a new variable and adds any value that we need to it. In our case, we set the SRC variable to the main.cpp
value. However, we can add more and more values to the same variable, as shown in this script:
SET(SRC main.cpp utils.cpp color.cpp )