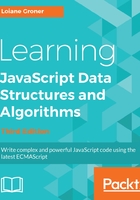
上QQ阅读APP看书,第一时间看更新
Declaring the spread and rest operators
In ES5, we can turn arrays into parameters using the apply() function. ES2015 has the spread operator (...) for this purpose. For example, consider the sum function we declared in the previous topic. We can execute the following code to pass the x, y, and z parameters:
let params = [3, 4, 5]; console.log(sum(...params));
The preceding code is the same as the code written in ES5, as follows:
console.log(sum.apply(undefined, params));
The spread operator (...) can also be used as a rest parameter in functions to replace arguments. Consider the following example:
function restParamaterFunction(x, y, ...a) { return (x + y) * a.length; } console.log(restParamaterFunction(1, 2, 'hello', true, 7));
The preceding code is the same as the following (also outputs 9 in the console):
function restParamaterFunction(x, y) { var a = Array.prototype.slice.call(arguments, 2); return (x + y) * a.length; } console.log(restParamaterFunction(1, 2, 'hello', true, 7));
The spread operator example can be executed at https://goo.gl/8equk 5, and the rest parameter example can be executed at https://goo.gl/LaJZqU.