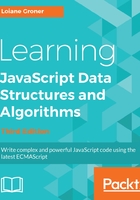
上QQ阅读APP看书,第一时间看更新
Scope variable
The scope refers to where in the algorithm we can access the variable (it can also be a function when we work with function scopes). There are local and global variables.
Let's look at an example:
var myVariable = 'global'; myOtherVariable = 'global'; function myFunction() { var myVariable = 'local'; return myVariable; } function myOtherFunction() { myOtherVariable = 'local'; return myOtherVariable; } console.log(myVariable); //{1} console.log(myFunction()); //{2} console.log(myOtherVariable); //{3} console.log(myOtherFunction()); //{4} console.log(myOtherVariable); //{5}
The above code can be explained as follows:
- Line {1} will output global because we are referring to a global variable.
- Line {2} will output local because we declared the myVariable variable inside the myFunction function as a local variable, so the scope will only be inside myFunction.
- Line {3} will output global because we are referencing the global variable named myOtherVariable that was initialized on the second line of the example.
- Line {4} will output local. Inside the myOtherFunction function, we referenced the myOtherVariable global variable and assigned the value local to it because we are not declaring the variable using the var keyword.
- For this reason, line {5} will output local (because we changed the value of the variable inside myOtherFunction).
You may hear that global variables in JavaScript are evil, and this is true. Usually, the quality of JavaScript source code is measured by the number of global variables and functions (a large number is bad). So, whenever possible, try avoiding global variables.