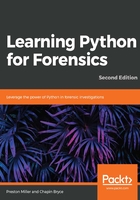
Designing the main() function
The main() function, defined on line 39, is fairly straightforward in this scenario. This function handles initial variable assignments and setup before calling parse_setup(). In the following code block, we create a docstring, surrounded with three double quotes where we document the purpose of the function, along with the data returned by it, as shown on lines 40 through 43. Pretty sparse, right? We'll enhance our documentation as we proceed as things might change drastically this early in development:
039 def main():
040 """
041 Primary controller for script.
042 :return: None
043 """
After the docstring, we hardcode the path to the setupapi.dev.log file on line 45. This means that our script can only function correctly if a log file with this name is located in the same directory as our script:
045 file_path = 'setupapi.dev.log'
On lines 48 through 50, we print our script information, including name and version, to the console, which notifies the user that the script is running. In addition, we print out 22 equal signs to provide a visual distinction between the setup information and any other output from the script:
047 # Print version information when the script is run
048 print('='*22)
049 print('SetupAPI Parser, v', __date__)
050 print('='*22)
Finally, on line 51, we call our next function to parse the input file. This function expects a str object that represents the path to the setupapi.dev.log. Though it may seem to defeat the purpose of a main() function, we will place the majority of the functionality in a separate function. This allows us to reuse code that's dedicated to the primary functionality in other scripts and for the main() function to act more as a primary controller. An example of this will be shown in the final iteration of this code. See the following line of code:
051 parse_setupapi(file_path)